Mysql2使用连接池对高频语句预处理以提升性能。
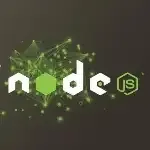
发布于2023-10-17 15:44 阅读1530次 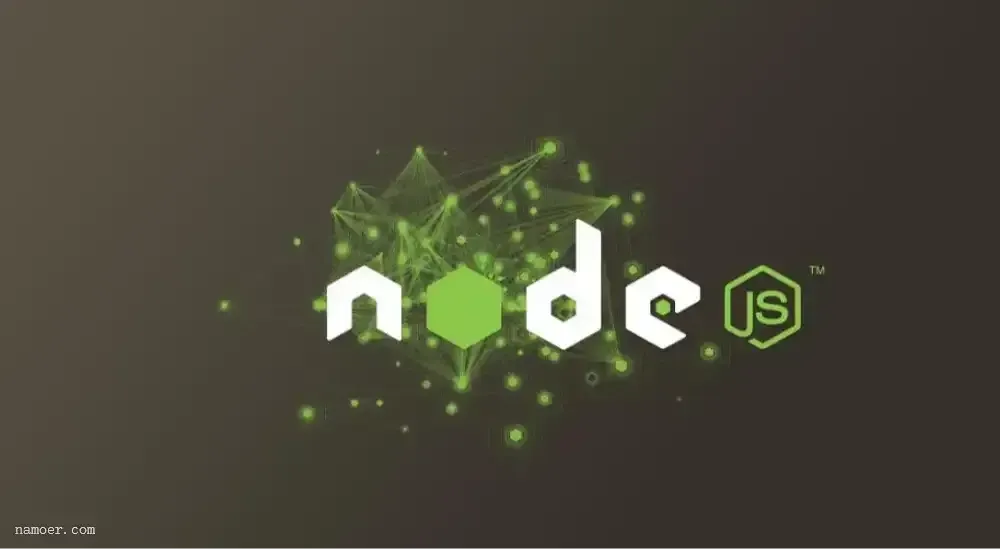
Mysql2适用于Node.js的MySQL客户端,专注于性能优化。支持SQL预处理、非UTF-8编码支持、二进制文件编码支持、压缩和SSL等等,这里主要记录一下使用预处理功能。
使用 MySQL2预处理的使用,可以提前准备好SQL预处理语句。 使用准备好的SQL预处理语句,MySQL 不必每次都为相同的查询做准备,这会带来更好的性能。使得高频查询语句的查询速度和性能得到大大提升。
连接池通过重用以前的连接来帮助减少连接到 MySQL 服务器所花费的时间,当你完成它们时让它们保持打开而不是关闭。这改善了查询的延迟,因为您避免了建立新连接所带来的所有开销。
**1、安装mysql2**
```
npm install mysql2
```
**2、定义数据库连接**
```
const mysqlConfig={
host:'127.0.0.1',
user:'root',
password:'password',
database:'database',
charset:'UTF8MB4_GENERAL_CI',
port:3306,
waitForConnections: true,
connectionLimit: 10,
queueLimit: 0
},
```
**3、创建连接池,使用Promise异步返回数据,并在开发模式下返回错误!**
```
import mysql from 'mysql2';
const pool=mysql.createPool(app.config.mysqlConfig);
export default (sql,params=[])=>{
params.forEach((item,index,self)=>{
if(typeof(item)=='string') self[index]=app.filterStr(self[index]);
});
//手动选择连接池使用后进行释放 可对连接池错误进行处理
return new Promise((resolve,reject)=>{
pool.getConnection((err, conn)=>{
if(err){
pool.releaseConnection(conn);
if(process.env.NODE_ENV == 'development') console.log(err);//开发模式 显示错误
reject(false);
}
pool.execute(sql,params,(err,res)=>{// execute将在内部调用prepare和query,短时内多次相同查询,直接调缓存,提升性能和查询速度
pool.releaseConnection(conn);
if(err){
if(process.env.NODE_ENV == 'development') console.log(err);//开发模式 显示错误
reject(false);
}else{
resolve(res);
}
});
});
});
}
```
**注:可以使用以下方式监听连接池进程:**
```
pool.on('acquire', connection=>{
console.log(获取数据库连接 [${connection.threadId}]);
});
pool.on('connection', connection=>{
console.log(创建数据库连接 [${connection.threadId}]);
});
pool.on('enqueue', connection=>{
console.log('正在等待可用数据库连接');
});
pool.on('release', connection=>{
console.log(数据库连接 [${connection.threadId}] 已释放);
});
```